It knows about Gson annotations, and has tons of options, although the defaults are sensible. If the node isnt an Object, Gson will throw an IllegalStateException, and if we attempt to .get() a node that doesnt exist Gson will return null and we have to handle possible NullPointerExceptions ourselves. com.google.gson.JsonSyntaxException:java.lang.IllegalStateException:BEGIN_12BEGIN_,json,Json Enthusiasm for technology & like learning technical. The algorithm which computes this JsonPatch currently generates following operations as per RFC 6902 -. To overcome this and compare JSON data semantically, we need to load the data into a structure in memory that's not affected by things like whitespace or by the order of an object's keys. or Compare or try some sample data or How can I pretty-print JSON in a shell script? For simple queries, the tree model can serve you well but its hard to avoid mixing up JSON parsing and application logic which can make the code hard to maintain. "Entries only on the left\n--------------------------", "\n\nEntries only on the right\n--------------------------", "\n\nEntries differing\n--------------------------". Please correct the
You need to register the InstanceCreator of a java class type with Gson first before using it. So comparing by position or as unordered items are alternative approaches to be applied depending on the interpretation of the array data. Under the hood, the JSONObject. Why do many companies reject expired SSL certificates as bugs in bug bounties? All you need to do is to use setVersion() method of GsonBuilder. would be much better represented for comparison purposes as: It may not look quite so natural, but the corresponding contacts will be aligned properly. JsonObject doesnt implement Iterable, so an extra call to .entrySet() is needed for the for loop. Assume we have two strings, representing the same JSON data, but one of them has some extra spaces at the end of it: Although the content of the JSON objects is equal, comparing the above as strings will show a difference: The same would happen if the order of keys in an object was varied, even though JSON is not usually sensitive to this: This is why it's we'd benefit from using a JSON processing library to compare JSON data. Goals for Gson Any object that has a unique key member should ideally be represented as an object where the key is pulled out as the member string this leads to unambiguous comparison. I dont consider this a huge loss though as it usually doesnt save a lot of code and there are more flexible alternatives. Can I tell police to wait and call a lawyer when served with a search warrant? Gson and Jackson from a performance point of view: Code Wrapper. There are three ways to model a date using the data types that JSON has: convert it into a number, a string, or a structured object. In another way, it can used to convert the JSON into equivalent java objects. In this tutorial we will learn how to calculate the difference between two JSON objects, in C#. If you are working with the same format of JSON a lot, the investment of creating classes is likely to be well worth it. Compare 2 Random Json String and return the difference as map. Objectsalso compare well in that each member property is identified by a string which should be unique within the object (it does not have to be unique but behaviour is unpredictable if they are not unique!). Note that if you care about the order of the elements, Json doesn't preserve order on the fields of Object s, so this method won't show those comparisons. Therefore corresponding members can be identified without ambiguity even if the order of the members is different. Instantly share code, notes, and snippets. Maven Dependency Let's add the dependency for the Gson library: However, if the array is being used as an unordered set of numbers, then the arrays should be considered equal. To parse json to object, use fromJson() method. Gson offers another mode of operation called data binding, where JSON is parsed directly into objects of your design. Can Martian regolith be easily melted with microwaves? Refresh the page, check Medium 's site status, or find something interesting to read. In this post we will compare two famous JSON specific API i.e. It's okay. We can compare it to another in a different order: Unlike JsonObject, JsonArrays equals method is order-sensitive, so these arrays are not equal, which is semantically correct: As we saw earlier, JsonParser can parse the tree-like structure of JSON. Why does Mister Mxyzptlk need to have a weakness in the comics? Ill be showing how answer the following questions in Java: Gson allows you to read JSON into a tree model: Java objects that represent JSON objects, arrays and values. val . JSON Formatter, JSON Validator, JSON Editor, JSON Viewer, JSON to XML, JSON to CSV, JSON to YAML, JSON Tree View, JSON Pretty Print, JSON Parser. Modeling date and time in JSON. Then save it to a new third object. To learn more, see our tips on writing great answers. Many times we need to compare specific portions of JSON Objects or JSON Arrays. Then save it to a new third object. Read more here about what information the resulting MapDifference object contains. The most popular Java libraries for working with JSON, as measured by usage in maven central and GitHub stars, are Jackson and Gson. Usually I find it does 90% of the work for me, but the classes often need some finessing once theyre generated. To use it for this project I removed all but one of the NEOs and selected the following options: The NeoWS JSON has a slightly awkward (but not unusual) feature - some data is stored in the keys rather than the values of the JSON objects. java.javax.JsonObjectGson public static class JavaxJsonObjConverter implements JsonSerializer lt JsonObject gt , JsonDeserialize . The three literal names, true, false and null are not a problem, though note they must be lower case. JsonParser is thread-safe so its OK to use the same one in multiple places. Get the source code . Your email address will not be published. How Intuit democratizes AI development across teams through reusability. Basically, instead of keeping . Android GSONJSON,android,gson,Android,Gson, public static class MyPojoImpl { @SerializedName("myNumber") private int myNumber; @SerializedName("myType") private String myType; @Override public List<String> getMyNumber() { return myNumber; } The default JSON output that is provide by Gson is a compact JSON format. For more complex queries, and especially when your JSON parsing is part of a larger application, I recommend data binding. But, if you found any problem using a class having no-args constructor, you can use InstanceCreator support. Here we can decide if the order of the elements from the JSONs matters or not. But the above should get you well on . java.lang.Character.equals () Java. So it is arrays that cause most problems in comparing JSON data. Just loop through each field in the second object, and if it's not present in the first or the value is different than the first, put that field in the return object. Connect and share knowledge within a single location that is structured and easy to search. Each JsonObject and JsonArraycontain other JsonElement objects, which can, themselves be of type JsonObject or JsonArray. class, new MyDeserializer ()) . See the example below. rev2023.3.3.43278. Suppose we have two strings, representing simple JSON objects, where the order of keys is different: The first object has fullName earlier than age: In this case, the JsonParser returns a JsonObject, whose equals implementation is not order-sensitive. And if you're looking for distraction-free mode, simply click the . It's not too hard to create a function like this. In order to save memory resources, you can create a Gson TypeAdapter that can work with JSON streams (and that is the base for every (de)serializer in JSON). June 16, 2022 To Compare two JSON objects need to run a for loop over the first object and check whether the second one has it or not in JavaScript. How can we prove that the supernatural or paranormal doesn't exist? Best and Secure Online JSON Compare Online work well in Windows, Mac, Linux, Chrome, Firefox, Safari, and Edge. well, you'll have to figure that out. There are some other java libraries also capable of doing this conversion, but Gson stands among very few which do not require any pre-annotated java classes OR sourcecode of java classes in any way. Bumps gson from 2.8.0 to 2.8.9. This is done by creating a class which extends TypeAdaptor
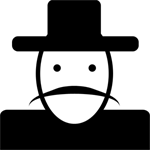