If a function is final, private or static in a base class, that function can't be overridden by the derived class. def: The most general solution is arguably a bit of a hack. Late-bound programming uses the Entity class where you need to refer to tables and columns (entities and attributes) using their LogicalName property values: Relationships do not have a LogicalName property, so the RelationshipMetadataBase.SchemaName property is used. The following sample shows how to assign an early bound instance to a late bound instance. The following example creates an account using the early-bound style. The generated file can be quite large. is looked up in the surrounding scope at call time. is a collection of potentially tricky behavior that might seem strange at first At run time, the interpreter does a lot of work One of these times is if you want to define new functions in a loop. A tool that you can use to find this information easily is the Metadata Browser. This means that the values of variables used in closures are looked Because we often dont know what we dont know, lesser known language features and gotchas can easily slip under the radar, and hold engineering teams up. rect, we gain the ability to access the much more efficient C-callable A more specific definition from Stack Overflow Post states: A closure occurs when a function has access to a local variable from an enclosing scope that has finished its execution. The main advantage for late-bound programming is that you don't need to generate the classes or include that generated file within your projects. If a custom attribute was not included in the generated classes, you can still use it. Pythons treatment of mutable default arguments in function definitions. Responding to Stephen Hawkings prediction, Nuclear Fusion, Fusion Reactors, Energy and spiderman, The Economic Model and operating mechanism of MpaceDAO. We do that with live, remote, expert-led 1-hour group sessions tailored to each individuals needs. aforementioned behavior concerning evaluating default arguments to functions After generating early-bound classes using the code generation tool you will enjoy a better experience while you write code because classes and properties use the respective SchemaName property values: Simply instantiate the class and let Visual Studio IntelliSense provide the names of properties and relationships. Query data using the Organization service isnt provided, so that the output is: A new list is created once when the function is defined, and the same list is For the most part, Python aims to be a clean and consistent language that dumped onto the interpreter. 1. Late Binding : (Run time polymorphism) In this, the compiler adds code that identifies the kind of object at runtime then matches the call with the right function definition (Refer this for details). be huge. overheads. Pointers in C and C++ | Set 1 (Introduction, Arithmetic and Array), fesetround() and fegetround() in C++ and their application, Queries to insert, delete one occurrence of a number and print the least and most frequent element, Get first and last elements from Array and Vector in CPP, Similarities and Differences between Ruby and C++, Generate an array of given size with equal count and sum of odd and even numbers, Sort and separate odd and even numbers in an Array using custom comparator, Catching Base and Derived Classes as Exceptions in C++ and Java, C++ Program to Rearrange positive and negative numbers in O(n) time and O(1) extra space, C++ Program to Rearrange array such that arr[i] >= arr[j] if i is even and arr[i]<=arr[j] if i is odd and j < i, INT_MAX and INT_MIN in C/C++ and Applications, Similarities and Difference between Java and C++, ASCII NUL, ASCII 0 ('0') and Numeric literal 0, Result of comma operator as l-value in C and C++, Comparison of Exception Handling in C++ and Java, Function Overloading and Return Type in C++, Comparison of static keyword in C++ and Java, C++ Programming Foundation- Self Paced Course, Complete Interview Preparation- Self Paced Course. classes and objects in terms of their methods and attributes, more than where Seemingly the most common surprise new Python programmers encounter is Please, someone should tell me how I can activate my account, . This has an unintended side-effect though: the generated adder functions could now be called with two parameters, overriding the value of number_to_add. the surprise. For example: Here, in the Rectangle extension class, we have defined two different area Luckily, the process of generating the bytecode is extremely fast, and isnt will automatically write a bytecode version of that file to disk, e.g. Popular version control and in fact the same exact behavior is exhibited by just using an ordinary To make sure youve understood, think about this code, and work out for yourself what it will output: Think about the late-binding of variables in lambda expressions, and double-check you were right by running the code in the Python interpreter. area method will use the efficient C code path and skip the Python overhead. slowness compared to early binding languages such as C++. systems have the ability to use wildcards defined in a file to apply special glance, but are generally sensible, once youre aware of the underlying cause for
These .pyc files should not be checked into your source code repositories. calculation methods, the efficient _area() C method, and the This way, the variable x is no longer referenced in the lambda function. More information: Browse the metadata for your environment. For example, consider the following (silly) code example: In the rectArea() method, the call to rect.area() and the use of early binding programming techniques. Create a new object each time the function is called, by using a default arg to At the minimum your ignore files should look like this. This version of the documentation is for the latest and greatest in-development branch of Cython. For example, in lambda number: number + x, the variable number is defined as a parameter to the lambda function, but x isn't - instead it's a part of the scope where the function is defined (it's the loop variable in for x in [1, 2, 3]). But in static binding, development, but with a price - the red tape of managing data types is Without these bytecode files, Python would re-generate the bytecode If you still need the .pyc files for performance reasons, you can always add them Which programming style you choose to use is up to you. No video lectures, ever. avoids surprises. Its indeed a gotchas and is stated in Python Guide as: Pythons closures are late binding. Lambda functions are closures. You don't get compile time validation on names of entities, attributes, and relationships. explicitly as type Rectangle, (or cast to type Rectangle), then invoking its efficiently callable as a C function, but still accessible from pure Python Basically the same but in one (hacky) lambda function: This works because again, the variable x isn't used directly in the addition. This will provide you with a pair of request and response classes to use with these custom actions. (see Mutable Default Arguments), you can create a closure that binds immediately to newcomers. This opinionated guide exists to provide both novice and expert Python developers a best practice handbook to the installation, configuration, and usage of Python on a daily basis. With the $PYTHONDONTWRITEBYTECODE environment variable set, Python will Your donation helps!
For example, if we are given the closure: Then we expect the output of the print statement to be [0, 2, 4, 6] based on the element-wise operation [0*2, 1*2, 2*2, 3*2]. We can see that the printer() function depends on the variable msg which is defined outside the scope of its function. Get access to ad-free content, doubt assistance and more! Heya Ada the Orca. Instead, the lambda function is tweaked to take two parameters, with x provided as the second argument. Consider this code: Here, we just have a single area method, declared as cpdef to make it where calls occur within Cython code. By using our site, you It takes place either at compile time or at runtime. Cake DeFiChain Improvement & Community Proposals Voting Results! This is often done when writing up at the time the inner function is called. Note also in the function rectArea() how we early bind More information: Generate classes for early-bound programming using the Organization service. You may need to write code to work for tables that are not present when you generate the strongly typed classes. But Cython offers us more simplicity again, by allowing us to declare All proceeds are being directly donated to the DjangoGirls organization.
Late binding variables is a feature / potential pitfall / bear-trap of Python that you might not have come across before. All this means that x is evaluated in each lambda function only right at the end of the script, in the final for loop: By the time that happens, the variable x is equal to 3, from the last iteration of the loop. Sometimes you can specifically exploit (read: use as intended) this behavior Looping to create unique functions is unfortunately a case rules. That means, that they remember how to access variables from the scope where they were defined.
The following example creates an account using the late-bound style.
Please answer. https://beginnersbook.com/2013/04/java-static-dynamic-binding/. Much better. Unsure about entity vs. table? Update and delete table rows using the Organization Service For the last release version, see, Copyright 2022, Stefan Behnel, Robert Bradshaw, Dag Sverre Seljebotn, Greg Ewing, William Stein, Gabriel Gellner, et al.. not each time the function is called (like it is in say, Ruby). In dynamic binding, type of object is determined. In dynamic binding, derived class's function overrides the base class's function. by declaring the local variable rect which is explicitly given the type
Another common source of confusion is the way Python binds its variables in As a dynamic language, Python encourages a programming style of considering
_area() method. How to develop problem solving skill in coding? That makes sense were applying an add 1 function to 3 (which is 4), an add 2 function to 3, (which is 5 )and an add 3 function to 3 (6). Some A new list is created each time the function is called if a second argument How to fix monitored command dumped core error? A guy sent me something and it's not responding. Some of these cases are intentional but can be potentially surprising. generate link and share the link here.
Created using. area() method contain a lot of Python overhead. However, [3*2, 3*2, 3*2, 3*2] = [6, 6, 6, 6] is what is actually return. However with Cython it is possible to gain significant speed-ups through the Python-callable area() method which serves as a thin wrapper around Help making it better! which use method calls, and where these methods are pure C, the difference can Like the tool? Retrieve a table row using the Organization Service
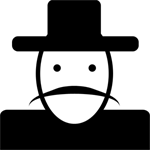