So the Coin Change problem has both properties (see this and this) of a dynamic programming problem. To learn more, see our tips on writing great answers. Follow the below steps to Implement the idea: Using 2-D vector to store the Overlapping subproblems. The row index represents the index of the coin in the coins array, not the coin value. Amount: 30Solutions : 3 X 10 ( 3 coins ) 6 X 5 ( 6 coins ) 1 X 25 + 5 X 1 ( 6 coins ) 1 X 25 + 1 X 5 ( 2 coins )The last solution is the optimal one as it gives us a change of amount only with 2 coins, where as all other solutions provide it in more than two coins. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide. Why recursive solution is exponenetial time? At first, we'll define the change-making problem with a real-life example. Your code has many minor problems, and two major design flaws. The specialty of this approach is that it takes care of all types of input denominations. Picture this, you are given an array of coins with varying denominations and an integer sum representing the total amount of money. There are two solutions to the Coin Change Problem , Dynamic Programming A timely and efficient approach. Will this algorithm work for all sort of denominations? Small values for the y-axis are either due to the computation time being too short to be measured, or if the number of elements is substantially smaller than the number of sets ($N \ll M$). We've added a "Necessary cookies only" option to the cookie consent popup, 2023 Moderator Election Q&A Question Collection, How to implement GREEDY-SET-COVER in a way that it runs in linear time, Greedy algorithm for Set Cover problem - need help with approximation. - the incident has nothing to do with me; can I use this this way? Note: Assume that you have an infinite supply of each type of coin. The dynamic programming solution finds all possibilities of forming a particular sum. table). The time complexity for the Coin Change Problem is O (N) because we iterate through all the elements of the given list of coin denominations. dynamicprogTable[coinindex][dynamicprogSum] = dynamicprogTable[coinindex-1][dynamicprogSum]; dynamicprogTable[coinindex][dynamicprogSum] = dynamicprogTable[coinindex-1][dynamicprogSum]+dynamicprogTable[coinindex][dynamicprogSum-coins[coinindex-1]];. return dynamicprogTable[numberofCoins][sum]; int dynamicprogTable[numberofCoins+1][5]; initdynamicprogTable(dynamicprogTable); printf("Total Solutions: %d",solution(dynamicprogTable)); Following the implementation of the coin change problem code, you will now look at some coin change problem applications. What can a lawyer do if the client wants him to be acquitted of everything despite serious evidence? A greedy algorithm is an algorithmic paradigm that follows the problem solving heuristic of making the locally optimal choice at each stage with the intent of finding a global optimum. Kalkicode. In this case, you must loop through all of the indexes in the memo table (except the first row and column) and use previously-stored solutions to the subproblems. Solve the Coin Change is to traverse the array by applying the recursive solution and keep finding the possible ways to find the occurrence. Once we check all denominations, we move to the next index. *Lifetime access to high-quality, self-paced e-learning content. Time Complexity: O(M*sum)Auxiliary Space: O(M*sum). Complexity for coin change problem becomes O(n log n) + O(total). Also, we assign each element with the value sum + 1. Hello,Thanks for the great feedback and I agree with your point about the dry run. How does the clerk determine the change to give you? The first column value is one because there is only one way to change if the total amount is 0. However, if the nickel tube were empty, the machine would dispense four dimes. Because there is only one way to give change for 0 dollars, set dynamicprog[0] to 1. If all we have is the coin with 1-denomination. The optimal number of coins is actually only two: 3 and 3. Since everything between $1$ and $M$ iterations may be needed to find the sets that cover all elements, in the mean it may be $M/2$ iterations. Minimising the environmental effects of my dyson brain. The convention of using colors originates from coloring the countries of a map, where each face is literally colored. Now that you have grasped the concept of dynamic programming, look at the coin change problem. Using the memoization table to find the optimal solution. Making statements based on opinion; back them up with references or personal experience. In other words, we can use a particular denomination as many times as we want. Buying a 60-cent soda pop with a dollar is one example. The quotient is the number of coins, and the remainder is what's left over after removing those coins. A greedy algorithm is the one that always chooses the best solution at the time, with no regard for how that choice will affect future choices.Here, we will discuss how to use Greedy algorithm to making coin changes. By using our site, you The main limitation of dynamic programming is that it can only be applied to problems divided into sub-problems. So the problem is stated as we have been given a value V, if we want to make change for V Rs, and we have infinite supply of { 1, 2, 5, 10, 20} valued coins, what is the minimum number of coins and/or notes needed to make the change? Initialize a new array for dynamicprog of length n+1, where n is the number of different coin changes you want to find. The above solution wont work good for any arbitrary coin systems. Next, we look at coin having value of 3. What sort of strategies would a medieval military use against a fantasy giant? $\mathcal{O}(|X||\mathcal{F}|\min(|X|, |\mathcal{F}|))$. Do you have any questions about this Coin Change Problem tutorial? The best answers are voted up and rise to the top, Not the answer you're looking for? This is due to the greedy algorithm's preference for local optimization. This article is contributed by: Mayukh Sinha. For example, for coins of values 1, 2 and 5 the algorithm returns the optimal number of coins for each amount of money, but for coins of values 1, 3 and 4 the algorithm may return a suboptimal result. Input: sum = 4, coins[] = {1,2,3},Output: 4Explanation: there are four solutions: {1, 1, 1, 1}, {1, 1, 2}, {2, 2}, {1, 3}. This is my algorithm: CoinChangeGreedy (D [1.m], n) numCoins = 0 for i = m to 1 while n D [i] n -= D [i] numCoins += 1 return numCoins time-complexity greedy coin-change Share Improve this question Follow edited Nov 15, 2018 at 5:09 dWinder 11.5k 3 25 39 asked Nov 13, 2018 at 21:26 RiseWithMoon 104 2 8 1 coin change problem using greedy algorithm. How do you ensure that a red herring doesn't violate Chekhov's gun? An amount of 6 will be paid with three coins: 4, 1 and 1 by using the greedy algorithm. For the complexity I looked at the worse case - if. The nature of simulating nature: A Q&A with IBM Quantum researcher Dr. Jamie We've added a "Necessary cookies only" option to the cookie consent popup. The function C({1}, 3) is called two times. Hi, that is because to make an amount of 2, we always need 2 coins (1 + 1). Answer: 4 coins. \text{computation time per atomic operation} = \text{cpu time used} / (M^2N). . It is a knapsack type problem. That can fixed with division. Follow the steps below to implement the idea: Sort the array of coins in decreasing order. How can I check before my flight that the cloud separation requirements in VFR flight rules are met? The valued coins will be like { 1, 2, 5, 10, 20, 50, 100, 500, 1000}. Saurabh is a Software Architect with over 12 years of experience. Here is the Bottom up approach to solve this Problem. My initial estimate of $\mathcal{O}(M^2N)$ does not seem to be that bad. Connect and share knowledge within a single location that is structured and easy to search. It has been proven that an optimal solution for coin changing can always be found using the current American denominations of coins For an example, Lets say you buy some items at the store and the change from your purchase is 63 cents. Solution: The idea is simple Greedy Algorithm. The Idea to Solve this Problem is by using the Bottom Up(Tabulation). Follow the steps below to implement the idea: Below is the implementation of above approach. For example, if we have to achieve a sum of 93 using the above denominations, we need the below 5 coins. Using indicator constraint with two variables. JavaScript - What's wrong with this coin change algorithm, Make Greedy Algorithm Fail on Subset of Euro Coins, Modified Coin Exchange Problem when only one coin of each type is available, Coin change problem comparison of top-down approaches. The diagram below depicts the recursive calls made during program execution. Time complexity of the greedy coin change algorithm will be: For sorting n coins O(nlogn). Disconnect between goals and daily tasksIs it me, or the industry? optimal change for US coin denominations. The coin of the highest value, less than the remaining change owed, is the local optimum. The fact that the first-row index is 0 indicates that no coin is available. The above problem lends itself well to a dynamic programming approach. Hence, we need to check all possible combinations. Why are Suriname, Belize, and Guinea-Bissau classified as "Small Island Developing States"? Else repeat steps 2 and 3 for new value of V. Input: V = 70Output: 5We need 4 20 Rs coin and a 10 Rs coin. Why Kubernetes Pods and how to create a Pod Manifest YAML? Using coins of value 1, we need 3 coins. # Python 3 program # Greedy algorithm to find minimum number of coins class Change : # Find minimum coins whose sum make a given value def minNoOfCoins(self, coins, n . Making statements based on opinion; back them up with references or personal experience. This post cites exercise 35.3-3 taken from Introduction to Algorithms (3e) claiming that the (unweighted) set cover problem can be solved in time, $$ How to use the Kubernetes Replication Controller? For an example, Lets say you buy some items at the store and the change from your purchase is 63 cents. I.e. How can I find the time complexity of an algorithm? Next, index 1 stores the minimum number of coins to achieve a value of 1. You will now see a practical demonstration of the coin change problem in the C programming language. S = {}3. And using our stored results, we can easily see that the optimal solution to achieve 3 is 1 coin. Can Martian regolith be easily melted with microwaves? Now, take a look at what the coin change problem is all about. Actually, we are looking for a total of 7 and not 5. As a result, dynamic programming algorithms are highly optimized. The greedy algorithm will select 3,3 and then fail, whereas the correct answer is 3,2,2. Problem with understanding the lower bound of OPT in Greedy Set Cover approximation algorithm, Hitting Set Problem with non-minimal Greedy Algorithm, Counterexample to greedy solution for set cover problem, Time Complexity of Exponentiation Operation as per RAM Model of Computation. Dynamic Programming is a programming technique that combines the accuracy of complete search along with the efficiency of greedy algorithms. I claim that the greedy algorithm for solving the set cover problem given below has time complexity proportional to $M^2N$, where $M$ denotes the number of sets, and $N$ the overall number of elements. Today, we will learn a very common problem which can be solved using the greedy algorithm. The problem at hand is coin change problem, which goes like given coins of denominations 1,5,10,25,100; find out a way to give a customer an amount with the fewest number of coins. The dynamic approach to solving the coin change problem is similar to the dynamic method used to solve the 01 Knapsack problem. Subtract value of found denomination from V.4) If V becomes 0, then print result. After understanding a coin change problem, you will look at the pseudocode of the coin change problem in this tutorial. Why do small African island nations perform better than African continental nations, considering democracy and human development? For example. vegan) just to try it, does this inconvenience the caterers and staff? Basically, here we follow the same approach we discussed. The difference between the phonemes /p/ and /b/ in Japanese. The complexity of solving the coin change problem using recursive time and space will be: Time and space complexity will be reduced by using dynamic programming to solve the coin change problem: PMP, PMI, PMBOK, CAPM, PgMP, PfMP, ACP, PBA, RMP, SP, and OPM3 are registered marks of the Project Management Institute, Inc. After that, you learned about the complexity of the coin change problem and some applications of the coin change problem. Time Complexity: O(V).Auxiliary Space: O(V). Use MathJax to format equations. Solution for coin change problem using greedy algorithm is very intuitive. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. While loop, the worst case is O(total). Refering to Introduction to Algorithms (3e), page 1119, last paragraph of section A greedy approximation algorithm, it is said, a simple implementation runs in time Hence, the minimum stays at 1. Whats the grammar of "For those whose stories they are"? Coin Change By Using Dynamic Programming: The Idea to Solve this Problem is by using the Bottom Up Memoization. Thanks to Utkarsh for providing the above solution here.Please write comments if you find anything incorrect, or you want to share more information about the topic discussed above. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. Does ZnSO4 + H2 at high pressure reverses to Zn + H2SO4? i.e. Trying to understand how to get this basic Fourier Series. For example, if you want to reach 78 using the above denominations, you will need the four coins listed below. Optimal Substructure To count total number solutions, we can divide all set solutions in two sets. Time complexity of the greedy coin change algorithm will be: While loop, the worst case is O(total). Approximation Algorithms, Vazirani, 2001, 1e, p.16, Algorithm 2.2: Let $\alpha = \frac{c(S)}{|S - C|}$, i.e., the cost-effectiveness of Asking for help, clarification, or responding to other answers. Okay that makes sense. I have the following where D[1m] is how many denominations there are (which always includes a 1), and where n is how much you need to make change for. The Coin Change Problem is considered by many to be essential to understanding the paradigm of programming known as Dynamic Programming. Iterate through the array for each coin change available and add the value of dynamicprog[index-coins[i]] to dynamicprog[index] for indexes ranging from '1' to 'n'. The function should return the total number of notes needed to make the change. For example, consider the following array a collection of coins, with each element representing a different denomination. At the worse case D include only 1 element (when m=1) then you will loop n times in the while loop -> the complexity is O(n). The space complexity is O (1) as no additional memory is required. However, we will also keep track of the solution of every value from 0 to 7. Connect and share knowledge within a single location that is structured and easy to search. Similarly, if the value index in the third row is 2, it means that the first two coins are available to add to the total amount, and so on. Since the smallest coin is always equal to 1, this algorithm will be finished and because of the size of the coins, the number of coins is as close to the optimal amount as possible. Update the level wise number of ways of coin till the, Creating a 2-D vector to store the Overlapping Solutions, Keep Track of the overlapping subproblems while Traversing the array. (we do not include any coin). Is time complexity of the greedy set cover algorithm cubic? Using 2-D vector to store the Overlapping subproblems. overall it is much . Finally, you saw how to implement the coin change problem in both recursive and dynamic programming. How can we prove that the supernatural or paranormal doesn't exist? Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. If you are not very familiar with a greedy algorithm, here is the gist: At every step of the algorithm, you take the best available option and hope that everything turns optimal at the end which usually does. Is it suspicious or odd to stand by the gate of a GA airport watching the planes? Also, once the choice is made, it is not taken back even if later a better choice was found. The main change, however, happens at value 3. The idea is to find the Number of ways of Denominations By using the Top Down (Memoization). If you do, please leave them in the comments section at the bottom of this page. Euler: A baby on his lap, a cat on his back thats how he wrote his immortal works (origin?). Also, each of the sub-problems should be solvable independently. Following is the DP implementation, # Dynamic Programming Python implementation of Coin Change problem. What is the bad case in greedy algorithm for coin changing algorithm? When amount is 20 and the coins are [15,10,1], the greedy algorithm will select six coins: 15,1,1,1,1,1 when the optimal answer is two coins: 10,10. . Making statements based on opinion; back them up with references or personal experience. Why does the greedy coin change algorithm not work for some coin sets? Are there tables of wastage rates for different fruit and veg? Row: The total number of coins. Again this code is easily understandable to people who know C or C++. Initialize set of coins as empty. Critical idea to think! If m>>n (m is a lot bigger then n, so D has a lot of element whom bigger then n) then you will loop on all m element till you get samller one then n (most work will be on the for-loop part) -> then it O(m). Connect and share knowledge within a single location that is structured and easy to search. Why do many companies reject expired SSL certificates as bugs in bug bounties? With this, we have successfully understood the solution of coin change problem using dynamic programming approach. We have 2 choices for a coin of a particular denomination, either i) to include, or ii) to exclude. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. And that is the most optimal solution. Subtract value of found denomination from amount. Is there a proper earth ground point in this switch box? Input and Output Input: A value, say 47 Output: Enter value: 47 Coins are: 10, 10, 10, 10, 5, 2 Algorithm findMinCoin(value) Input The value to make the change. Also, we implemented a solution using C++. By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. Asking for help, clarification, or responding to other answers. Is it correct to use "the" before "materials used in making buildings are"? return solution(sol+coins[i],i) + solution(sol,i+1) ; printf("Total solutions: %d",solution(0,0)); 2. In this tutorial, we're going to learn a greedy algorithm to find the minimum number of coins for making the change of a given amount of money. "After the incident", I started to be more careful not to trip over things.
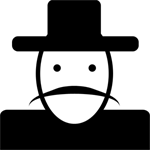